Open Web Technologies
Creating Web Games with Crafty.js
by Adrian Gaudebert
Web Developer for 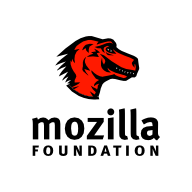
Today's planning
- Crafty.js ~20 min
- Preparations ~5 min
- Workshop ~90 min
Crafty.js
Code like a fox
What is it?
- Game Engine
- For the Web
- JavaScript
What does it do?
- Extensible architecture
- Game loop
- Basic elements
Crafty.init(STAGE_WIDTH, STAGE_HEIGHT);
A problem of inheritance
A problem of inheritance
A problem of inheritance
A solution with composition
A solution with composition
A solution with composition
Crafty.c('MyComponent', {
init: function () {
this.myVar = 0;
},
myComponent: function (myParam) {
this.myVar = myParam;
}
});
var enemy = Crafty.e('2D, Sprite, Armed, Moving');
var sheep = Crafty.e('2D, Sprite, Moving');
var tower = Crafty.e('2D, Sprite, Armed');
Baldur's Gate - Main menu
Baldur's Gate - Character creation
Baldur's Gate - In game
// Create a scene
Crafty.scene('my_scene', function () {
Crafty.e('2D, DOM, Text')
.attr({ x: 20, y: 20 })
.text('Hello!');
});
// And start the scene
Crafty.scene('my_scene');
// With Canvas
Crafty.canvas.init(); // after Crafty.init()
Crafty.e('Canvas, 2D, ...');
// With DOM
Crafty.e('DOM, 2D, ...');
Crafty.e('2D, DOM, Text')
.bind('EnterFrame', function () {
this.x += 1;
this.text = 'Frame number '
this.text += Crafty.frame();
});
A running Caveman
Crafty.sprite(34, 'img/caveman.png', {
'caveman1': [0, 0],
'caveman2': [1, 0],
'caveman3': [3, 3],
});
Crafty.e('2D, DOM, Sprite, caveman1')
.attr({ x: 50, y: 50 });
A running Caveman
Crafty.e('2D, DOM, SpriteAnimation, caveman1')
.attr({ x: 50, y: 50 })
.animate('walk', 0, 0, 3)
.animate('walk', 4, -1);
Crafty.e('2D, Keyboard')
.bind('EnterFrame', function () {
if (this.isDown('ARROW_RIGHT') ||
this.isDown('D')) {
this.x += 1;
}
});
Crafty.e('2D, Mouse')
.areaMap([
[0, 0], [0, 34],
[34, 34], [34, 0]
])
.bind('Click', function () {
console.log('CLICKED!!!11!1');
});
Twoway, Fourway, Multiway